Add Flap Animation
To animate the bird we'll need two new components. One to represent the sprite animation details, and another to manage the animation. These will be SpriteAnimationDetails
and SpriteAnimation
respectively.
Sprite Animation Details
SpriteAnimationDetails
is exactly the same as the SpriteData
class, with two new properties: frameWidth
and frameHeight
. Once we know the width and height of each frame within the animation, we can calculate how many images we have in that animation. We use the prefix frame
because the images that make up an animation are commonly referred to as frames.
We've talked about sprite data to represent something like a single complete image in the sprite sheet. Like the background. There's no reason the sample itself couldn't be made up of multiple images. This is exactly what we're going to do. We'll extend the sprite data object structure we know to represent a single sample of the sprite sheet, which itself has multiple images denoted by frameWidth
and frameHeight
.
Here's a diagram to help you visualize this:
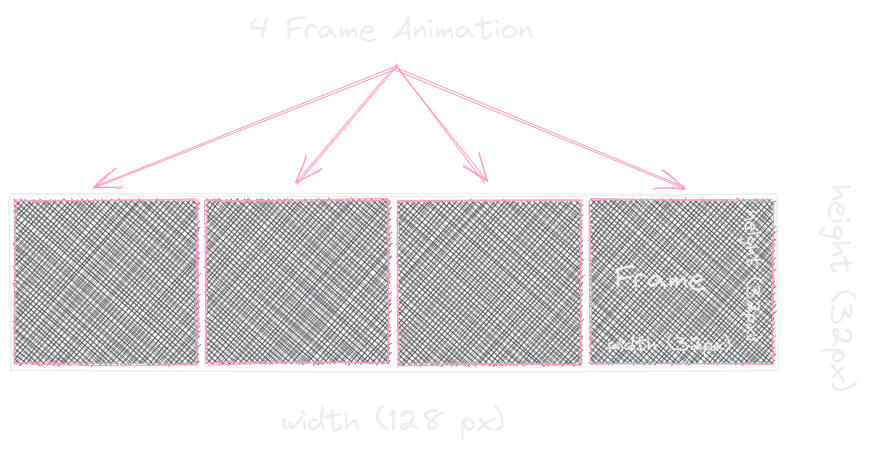
Create a new SpriteAnimationDetails
component:
Sprite Animation
We also need a class to manage the animation. We'll call this SpriteAnimation
.
Here's what this class will need to know at a high level:
- The duration (in seconds) of the entire animation
- The sprite animation details to determine how many frames there are, and what the duration of each frame will be
- Track the progress of the current frame, so we know when to move to the next frame
- The sprite data of the current frame we're on so we can render it
We're assuming that animations are only laid out horizontally, because that's all we need to handle our bird. If you want to support animations that are laid out both vertically and horizontally, you'll need to add some logic to handle that. We also assume the animation will loop.
Adding Animation to the Bird
We now need to:
- Update the
Bird
constructor to take aSpriteAnimation
instance - Add an
update()
method to the bird to update the animation - Draw the current animations frame in the
draw()
method - Start calling the
update()
method in the game loop
Now update the instance created in main.ts
to use the new SpriteAnimation
class:
We're leveraging the preconfigured spriteMap
here again to pull the frame data we need from the sprite sheet. We also set our animation duration to 0.3
seconds.
Then add bird.update()
within frame()
in main.ts
:
Now we're animating!
Next, we'll get the bird to flap upward on click.