Colliders
Circle Collider Component
For the circle collider we'll store the radius, and the position offset. The position offset would be used if we needed to adjust the position of the collider relative to the birds position. We won't be using offsets for the birds circle collider, but we will for pipes later on, so we'll include it for consistency.
The diagram below shows the relationship between the circle collider and the bird:
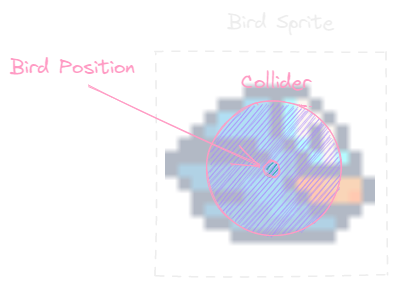
The collider is positioned directly at the birds position, and will also be slightly smaller than the bird visually. We say visually because the bird sprite is actually a rectangle, and would be much larger than the collider.
Create the cirlce collider:
Box Collider Component
The box collider component class will store the width, height and offset of the box. We'll want to adjust the position of the collider relative to the entity's position. This won't be true for the ground, but we will leverage the offset when we get to the pipes.