Colliders and Debug
Let's add some box colliders to our pipes and make sure we can see them when we toggle debug (press the d
key).
Box Colliders
Update the pipe entity to add a box collider:
We're applying our usual 50% opacity on the collider to make it easier to see the underlying sprite. We're applying some logic to set the colliders y
position based on the type
as well.
When you check the browser you should see the colliders after pressing the d
key.
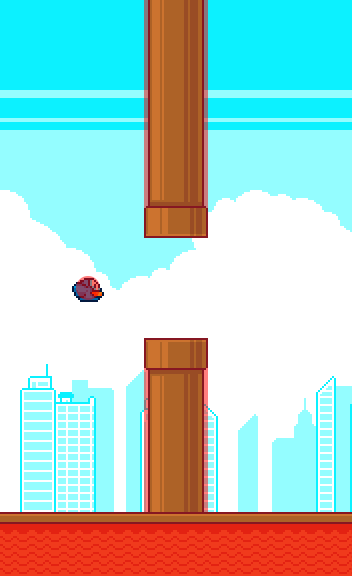
The collider is as wide as the pipe rim. Let's change that so the collider is a little more forgiving and matches the size of the pipe body.
Collider Sizing
We're going to store the pipe collider x offset
and the width
in our config:
The reason we store the x offset is to account for some of the transparent pixels in the pipe body slice sprite. These transparent pixels exist to make the pipe body sprite match the width of the pipe rim sprites.
Now we'll update the pipe entity to make use of this information:
Which will get us a slightly tighter collider:
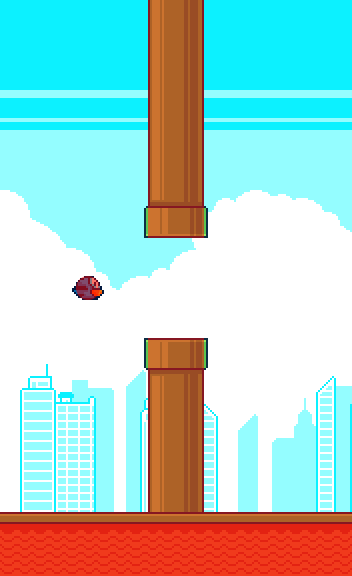
Much better! Let's move on to some clean up.